PYTHON PROGRAMMING
10

PART 5 – USING FUNCTIONS
PROGRAMS
PROGRAM 1 – SIMPLE FUNCTION
To discuss:
·
A
function implementation on its own doesn’t do anything.
·
Calling
a function means using a function.
·
We
call a function by using its name.
·
Functions
often have parameters that are passed to them.
def hey_there(name): print("Hey there", name, "my friend!") hey_there("Felix") hey_there("Leylah") |
PROGRAM 2 – RETURN STATEMENT
To discuss:
·
A
return statement allows a value to be returned from the function.
·
We
need to use the returned value when we call a function that returns a value.
def add(num1, num2, num3): return num1 + num2 + num3 sum = add(4, 5, 3) print(sum) |
PROGRAM 3A – RANDOM DICE ROLL
import random def d(sides): return random.randint(1, sides) print(d(5)) #rolling a d5 print(d(10)) #rolling a d10 |
PROGRAM 3B – BEST OF TWO DIE
def d(sides): return random.randint(1, sides) dice1 = d(6) dice2 = d(6) if dice1 >= dice2: print(dice1) else: print(dice2) |
PROGRAM 3C – THE MAX FUNCTION
import random def d(sides): return random.randint(1, sides) def maximum(val1, val2): if val1 >= val2: return val1 else: return val2 dice1 = d(6) dice2 = d(6) print(maximum(dice1, dice2)) |
Please clearly state at the top of your program if
you are doing option 1 or option 2.
TASK 1 – OPTION 1 (MAX OF 90%)
Use the
functions in the program above to roll a d10, two d20 and a d8. You should add the D10, the best of the
two D20s and the D8 together and output that total to screen.
SAMPLE IO
Your rolls:
D10: 5
D20: 17
D20: 4
D8: 7
Total: 29
|
TASK 1 – OPTION 2 (MAX OF 100%)
Write the
program that will roll three d20 die.
It will output the result of all three. Then, it will add up the best two die
results and output that total.
SAMPLE IO
Your rolls:
D20: 9
D20: 17
D20: 10
Best Two
Total: 27
|
PROGRAM 4 – ODD & EVEN FUNCTIONS
def is_even_number(num): if num % 2 == 0: return True else: return False def is_odd_number(num): if num % 2 == 1: return True else: return False print(is_even_number(5)) print(is_odd_number(5)) |
PROGRAM 5 – PRIMES
To discuss:
·
Functions
provide the opportunity to hide complexity.
·
Even
if we don’t know how a function is doing its calculation, we can still know
how to use it.
def is_prime_number(num): for f in range(2, num): if num % f == 0: return False return True print(is_prime_number(17)) |
TASK 2
a)
Use
Python to figure out the next prime number after the number 48. Note that you do not need to submit any
code for this task, you simply need the answer. So it is up to you to determine how you
want to use Python to solve this.
b)
Same
as in a), but find the next prime number after 1328.
|
PROGRAM 6 – AREA FUNCTIONS
def rectangle_area(length, width): return length * width print(rectangle_area(4, 8)) |
TASK 3
a)
Start
with the code above. Using the rectangle_area function as reference, create a triangle
area function.
b) Test your new function to make sure
that it works.
c)
Use
the two functions to calculate the entire area of the house below. You need to submit your code. You should put the answer as a comment at
the bottom of your code.
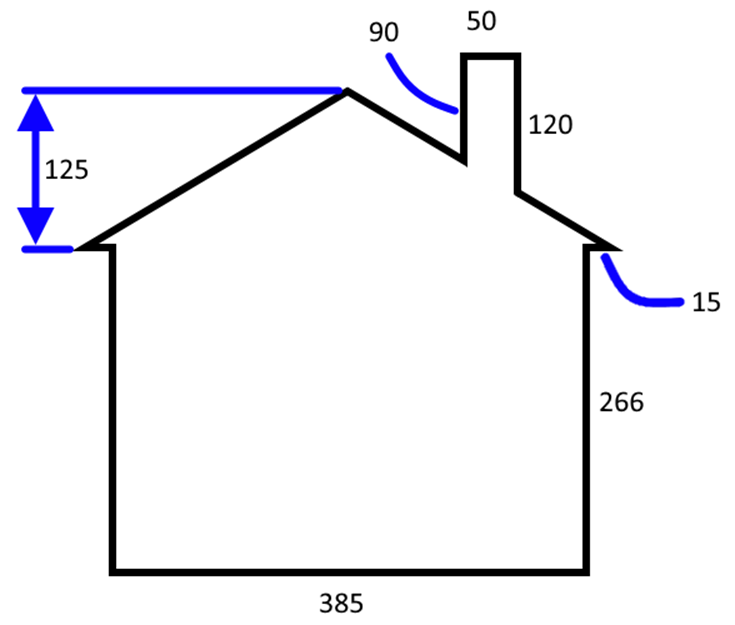
|

|