Java
OOP GUIDE / WORK

GRID CLASS SOLUTIONS

TASK –
PART 1 – TILE CLASS
Here is my solution:
public class
Tile
{
public int r, g, b;
public Tile(int r, int g, int b)
{
setColour(r,g,b);
}
public Tile()
{
setRandomColour();
}
public void setRandomColour()
{
r = (int)(Math.random() *
256);
g = (int)(Math.random() *
256);
b = (int)(Math.random() *
256);
}
public void setColour(int r, int g, int b)
{
this.r = r;
this.g = g;
this.b = b;
}
}
|

TASK –
PART 2 – PRACTICING WITH NOOPDRAW AND TILE
Here
is my solution:
public class TileTester
{
public static void
main(String[] args)
{
NOOPDraw.createWindow();
Tile t1 = new Tile();
NOOPDraw.setColor(t1.r, t1.g, t1.b);
NOOPDraw.fillRectangle(0, 0, 20, 20);
Tile t2 = new
Tile();
NOOPDraw.setColor(t2.r, t2.g, t2.b);
NOOPDraw.fillRectangle(20, 0, 20, 20);
Tile t3 = new
Tile();
NOOPDraw.setColor(t3.r, t3.g, t3.b);
NOOPDraw.fillRectangle(40,
0, 20, 20);
}
}
|
The
above code create the following window (image has
window resized for convenience):
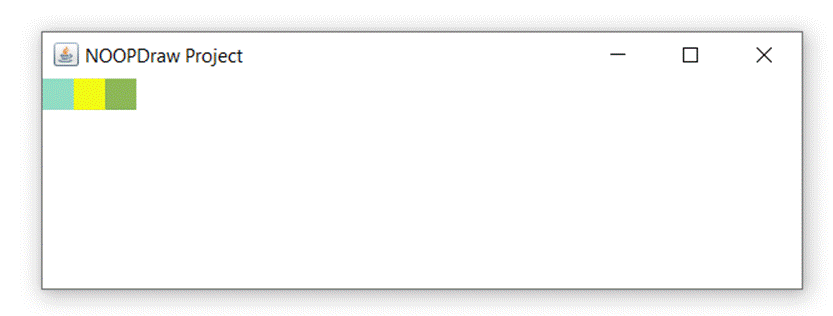

TASK –
PART 3 – THE GRID CLASS
Here
is my solution:
public class
Grid
{
public Tile[][] grid;
public int tileSize;
public Grid(int rows, int cols)
{
grid = new
Tile[rows][cols];
for (int r=0; r<rows; r++)
{
for (int c=0; c<cols; c++)
{
grid[r][c] = new Tile();
}
}
tileSize =
10;
}
//Pre-condition: NOOPDraw window must already exist.
public void draw()
{
for (int r=0; r<grid.length; r++)
{
for (int c=0; c<grid[r].length; c++)
{
Tile t = grid[r][c];
NOOPDraw.setColor(t.r, t.g, t.b);
NOOPDraw.fillRectangle(c*tileSize, r*tileSize, tileSize, tileSize);
}
}
}
}
|
|