EXAMPLE 1 – EMPTY
WINDOW
Here
is a simple window generated using Java FX:
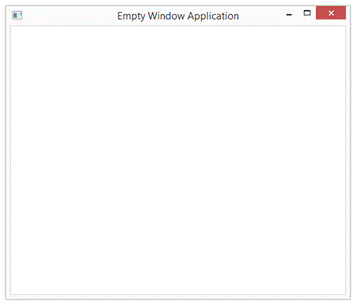
The
code to create the above window is shown below:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.FlowPane;
import javafx.stage.Stage;
public class EmptyWindow extends Application
{
@Override
public void start(Stage primaryStage)
throws Exception
{
FlowPane layout = new FlowPane();
Scene
scene = new
Scene(layout,500,400);
primaryStage.setScene(scene);
primaryStage.setTitle("Empty Window Application");
primaryStage.show();
}
public static void main(String[] args)
{
Application.launch(args);
}
}
|
CODE EXPLANATION –
IMPORT STATEMENTS
First,
you probably notice all of the import statements at the top. Thankfully, most IDEs (including Eclipse)
help us fill in the import statements whenever we use an unknown
class. However, one common error is
that students are given more than one choice for importing and they choose
a class that is not part of Java FX.
Take a good look at the import statements. They all start with "javafx". So
should all of yours.
CODE EXPLANATION –
EXTENDING APPLICATION
The
line that declares the class will always include "extends
Application". This means that
our class inherits all of the functionality of the Application class in
Java FX. As a consequence to
extending Application, we are forced to implement the method:
public void start(Stage primaryStage)
This
method is automatically called whenever we run an application using Java
FX. So, it is in this method that we
will place our statements.
CODE EXPLANATION –
MAIN FUNCTION
Most
IDEs can run Java FX applications without a main function included. However, to make sure that your program would
run in all IDEs, it is a good idea to include a basic main function that
will simply launch the Java FX application.
In
Eclipse, we need the main function to let Eclipse know which class to
run. However, after running the
application once, we can actually get rid of the main function all
together.
The
main function looks like this:
public static void
main(String[] args)
{
Application.launch(args);
}
The
launch function in Application does some advanced stuff to get the start
method to run. We won't focus on the
"how" of this. We just
care that it works at this point.
CODE EXPLANATION –
ORGANIZATION INSIDE THE START METHOD
The
entire window is referred to as a stage. The section that contains the content of
the window is called a scene.
Here
is the start method from above:
public void
start(Stage primaryStage) throws Exception
{
FlowPane layout = new FlowPane();
Scene
scene = new Scene(layout,500,400);
primaryStage.setScene(scene);
primaryStage.setTitle("Empty Window Application");
primaryStage.show();
}
The
start method automatically provides us with a reference to the stage. Our job is to insert the scene that we
want onto that stage. The stage will
mold with the scene's size.
A
scene needs to include some form of organization for its content. In the example above, we are using a FlowPane layout to force any content added to the scene
to flow one piece after the other.
More on this later. Just know
that you need some form of a layout object to create the scene. Also, many examples call this object root
instead of layout as it is the root to a tree of other objects.
So,after we create the FlowPane layout, we use it to create the scene. Then, we set the scene on the primary
stage. We also set the title of the
window and finally make the window visible using primaryStage.show();
|