INDIVIDUAL WORK
QUESTION #1
Is the
following array trace showing Insertion Sort, Selection Sort, Bubble Sort
or Merge Sort?
A)
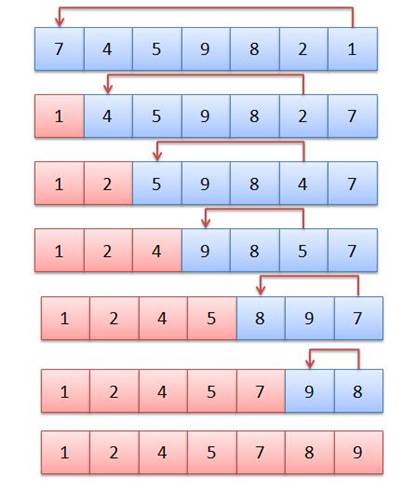
B)
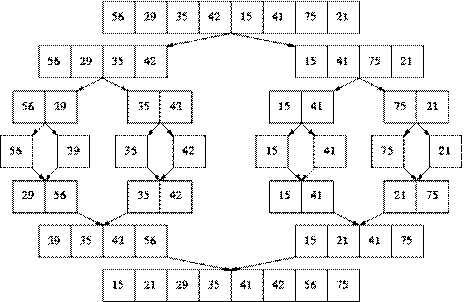
C)
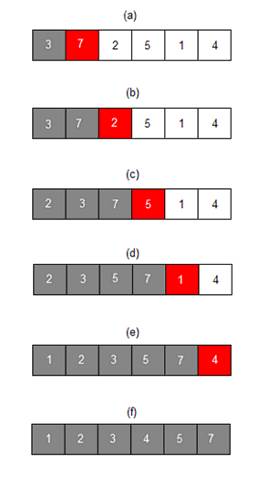
D)
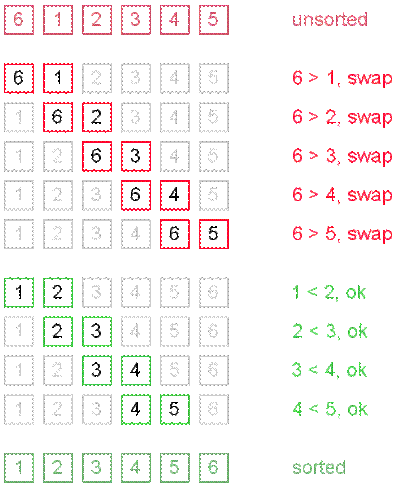
QUESTION #2
Consider
the array below:

a) Do an array trace of it being sorted
with Selection sort.
b) Do an array trace of it being sorted
with Insertion sort.
QUESTION #3
Implement
the following function:
public static
void swap (int[] a, int i,
int k)
The
function simply swaps the values at element i
with the value at element k.
|