INDIVIDUAL WORK
QUESTION #1 – FILL IN THE BLANKS (SELF-CHECK)
a)
The word pointer is another word for
______________.
b)
A null pointer exception is a(n) _____________
that occurs when you try to use an object that has not been created yet.
c)
The default value for integers in arrays is
_____________.
d)
The default value for all objects in arrays is
______________.
e)
What will the following code output?
String[] arr = new String[4];
System.out.println(arr[0]);
SOLUTION
Check your answers here.

QUESTION #2 – ARRAYS IN MEMORY (SELF-CHECK)
a) What will the
array look like in memory after the following statement?
Point[]
pts = new Point[6];
b) What will the array look like in memory after the following statement?
Point[] pts = {new
Point(7,3), new Point(0,4), new
Point(1,2)};
`
c) What will the array look like in memory after the following statement?
Point[] pts = new
Point[4];
pts[2]
= new Point(7,5);
d) Write the statement(s) required to create the following Point array in
memory.
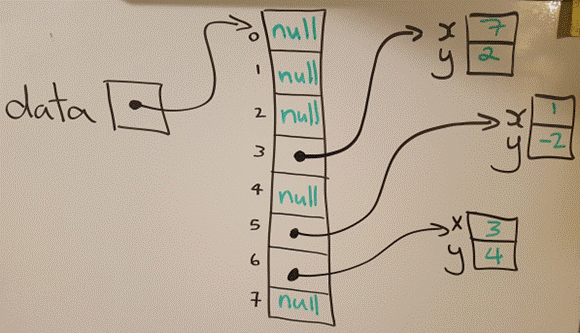
SOLUTION
Check your answers here.

QUESTION #3 – LOTSA
CLOWNS (SELF-CHECK)
Copy the
code from this Clown class to your project. Examine the Clown class to get an
understanding of it. Below are a few
questions to help you examine the Clown class.
PART A – OOP REVIEW
A)
How many instance variables does the class have?
B)
Are the instance variables accessible from other
classes?
C)
Once a Clown instance is created, is it possible
to change any of the instance variables?
D)
What line of code is required to create an
instance of Clown named bozo?
E)
What line of code is needed to output a String
representation of the Clown object to screen? Try it.
PART B – ARRAYS
F)
Write the statement(s) that create(s) a Clown
array that contains 8 clowns. Make
sure the Clown objects are created as well.
G)
Use a for loop to output the String
representation of each Clown instance in the array one at a time.
H)
Use another for loop to make all Clown instances
angry. Then, in yet another for
loop, output String representations of each Clown once again to make sure
they truly are angry clowns.
SOLUTION
Check your answers here.

QUESTION #4
Write a
function named totalDistance that gets a Point array and another Point q
object as parameter. It then returns
the total distance between q and all points in the array. This would be as if we want to draw lines
from q to all other points and want to know how long all lines add up
to.
Test your
function.

QUESTION #5
You are the
head of Amazon Canada. You want to
determine where is the best location to setup an airport to fly goods to
important cities. All cities are
Points on a map. Your airport has to
be at one of the cities. You want to
place it so that you minimize the amount of flying being done.
Use your
function from the previous question to create the function bestHubLocation
that gets a Point array as parameter and calculates the totalDistance using
each point as a hub. It then returns
the index of the best Point to choose as a hub.
Test your
program. It should output the Point
as well as the totalDistance to all other locations from that hub.
|