EXAMPLE (RECTANGLE CLASS)
In this example, we will create a Rectangle
class that will have four different constructors. Rectangles of this class are defined by
the x and y coordinates of their bottom left and top right points. So, there are four data fields named x1,
y1, x2, y2.
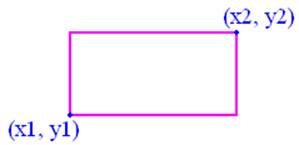
Here are the class guidelines:
·
It will have four double data fields named x1,
y1, x2, y2.
·
Constructor #1 has four double arguments, one
for each data field.
·
Constructor #2 has no arguments. It will create a Rectangle with opposite
corners (0,0) and (1,1).
·
Constructor #3 has two double arguments. The arguments will be the values for x2
and y2. The value of (x1, y1) will
simply be (0,0).
·
Constructor #4 has three double arguments. The first two arguments will be values
for x1 and y1. The third argument
will be both the height and the width of the rectangle (square). We have to calculate the value of x2 and
y2 based on this.
public class Rectangle
{
public double x1;
public double y1;
public double x2;
public double y2;
//CONSTRUCTOR #1
public Rectangle(double mx1, double my1, double mx2,
double my2)
{
x1 = mx1;
y1 = my1;
x2 = mx2;
y2 = my2;
}
//CONSTRUCTOR #2
public Rectangle()
{
x1 = 0.0;
y1 = 0.0;
x2 = 1.0;
y2 = 1.0;
}
//CONSTRUCTOR #3
public Rectangle(double mx2, double my2)
{
x1 = 0.0;
y1 = 0.0;
x2 = mx2;
y2 = my2;
}
//CONSTRUCTOR #4
public Rectangle(double
mx1, double my1, double side)
{
x1 = mx1;
y1 = my1;
x2 = mx1 + side;
y2 = my1 + side;
}
}
We can now create Rectangle objects using any of
the four constructors. Here is an
example of creating an object with each one of the constructors.
Rectangle r1 = new Rectangle(0.0, 8.0, 10.5, 9.0); //1st
constructor
Rectangle r2 = new Rectangle(); //2nd constructor
Rectangle r3 = new Rectangle(12.4, 8.2); //3rd
constructor
Rectangle r4 = new Rectangle(2.0, 3.0, 6.0); //4th
constructor
The object r1 would look like this in memory:
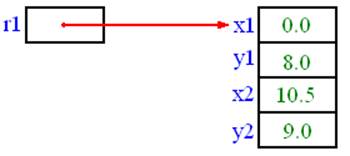
|