Java
TOPIC 36 – CONSTRUCTORS I

LESSON NOTE - REVIEW

ABOUT THIS UNIT
Object-oriented programming is a programming approach that involves the
grouping of associated data and functionality inside of objects. This then allows the programmer to think of
problems in terms of real world objects instead of a large series of
variables and separated operations.
In this unit,
we will look at new concepts relating to object-oriented programming
(OOP). This will include far more
details on constructing objects and adding functionality to objects. We will then also learn about protecting
data from taking on illegal values.
RECAP
We have
already created classes that contain only instance variables (also known as data fields). We have used
these classes to create objects (or instances).
EXAMPLE
Here is a simple Point class that contains two instance variables (data fields)
called x and y:
public class Point
{
public double x;
public double y;
}
We can now
create Point objects and access the instance variables (data fields).
public class Tester
{
public static void main(String[] args)
{
//Create
a Point object named p.
Point p = new Point();
//We can
set the values of the data fields of object p.
p.x = 5.0;
p.y = 7.2;
//We can
print the values of p by doing:
System.out.println("("
+ p.x + ", " + p.y
+ ")");
}
}
Executing the code above would output:
(5.0, 7.2)
|
REVISTING
TERMINOLOGY
In the example above, the class
name is Point. We created a Point object named p. The object p can also be referred to as an instance of the class Point.
The term object and the term instance can be used interchangeably.
CLASS
NAMING CONVENTION
Reminder: Classes should be named in the same way as
variables except the first character should always be an upper case
letter.
OBJECTS IN
MEMORY
Consider the following code:
Point p = new Point();
p.x =
5.0;
p.y = 6.1;
The above
code will create an object in memory.
The object p will be a memory reference to the location in memory
where the data fields are stored.
It will look like this:
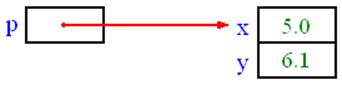

|