CHALLENGE
In this challenge, you will work with three different
classes. Two classes, CargoShip and SeaContainer,
will contain only data fields (instance variables) while the third will
contain your program code.
DETAILS
A sea container is simply a large
container that is used to store goods when they are transported on large
ships. They are usually 6 meters or 12
meters in length. When empty, they
weigh about 2 500 kg.
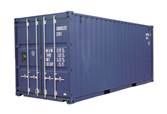
A
cargo ship is a ship that can
carry many sea containers from one location to another. They have a maximum number of containers
they can carry. They also have a
maximum weight that they can hold.
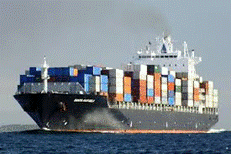
WORK
1 - Create a class called CargoShip that has the
following data fields (instance variables):
·
name – the name of the ship
·
origin – the country from which
the ship originates
·
containers – the number of
containers on the ship
·
maxContainers
– the max number of containers that the ship can hold
·
cargoWeight
– the weight of all the cargo
·
maxCargoWeight
– the max weight of cargo that the ship can hold
2 – Create a class called SeaContainer that has the
following data fields (instance variables):
·
id – this is simply the name of
the container (or an id number)
·
cargoWeight
– the weight of all the cargo
·
size – the size of the container
in container units (either 1 or 2)
Note:
A container that is of size 2 simply counts as two containers on the
boat. A 6m container is a size 1 while
a 12m container is a size 2.
We will assume that containers have no max cargo weight.
3 – Create a class called CargoProgram. Place the main method inside it. This class will contain your program
code.
ACTIONS
1-Create
an object that represents the following:
There is a cargo ship named "Paris on Water" that comes from
France. It can hold up to 400 sea
containers and a max cargo weight of 6 000 000 kg. It currently has 120 containers all
weighting 2500kg (empty).
2-Write
the output statements required to output the state of the ship like this:
Ship: Paris on Water (France)
Sea container capacity: 120 of 400
Weight capacity: 300000 of 6000000
Of course, everywhere possible, you need to make use
of variables to do the above output statements.
3-Create
the following three sea container objects:
Container
#1:
This container is 6m long. It weighs
7000kg.
Container #2:
This container is 6m long. It weighs
5000kg.
Container #3
This container is 12m long. It weighs
22000kg.
4
– Write the output statements needed to output the content of each
container. It should look like this:
Container 1
Size: 1
Weight: 7000kg
5 – Container 1 is loaded onto the ship.
Output the new state of the ship.
6 – Container 2 is loaded onto the ship.
Output the new state of the ship.
7 – Container 3 is loaded onto the ship.
Output the new state of the ship.
8
– Once back at sea, a container weighing 2500kg falls off the ship and is
lost. It was a single size
container. Output the new state of the
ship.
9 – At sea, another we encounter another ship described below. Create that object.
The Great Wall, from China, is overcapacity.
It has 121 sea containers despite only being designed for 120
containers. Its weight capacity is
sitting at 1500000 of 2000000kg.
10
– Output the state of the Great Wall ship.
11 – Having difficulties navigating over capacity, The Great Wall contacts
Paris on Water to unload one container.
Create the container object with the following info:
Container #4
This container is 6m long. It weighs 8500kg.
11 – Container #4 is transferred from The Great Wall to Paris on Water. Update both ships and output the state of
each one to screen.
SAMPLE OUTPUT
Here is partial sample output. Notice
that I included the STEP number to keep things organized.
STEP
2
Ship: Paris on Water (France)
Sea container capacity: 120 of 400
Weight capacity: 300000 of 6000000
STEP 4
Container 1
Size: 1
Weight: 7000kg
Container 2
Size: 1
Weight: 5000kg
Container 3
Size: 2
Weight: 22000kg
STEP 5
Ship: Paris on Water (France)
Sea container capacity: 121 of 400
Weight capacity: 307000 of 6000000
...
|
|